8 tools for every Java developer’s toolkit
Java developers have an enormous selection of libraries, utilities, and programs at their disposal. Each one has its merits, but there are a few that stand out from the crowd due to their popularity, versatility, and usefulness.
These eight tools cover the full gamut of Java development, from code building to bug squashing. Learning these tools can help you improve the quality of your code and become a more efficient Java developer.
Eclipse
Despite the increasing popularity of IntelliJ IDEA, NetBeans, and other IDEs, surveys indicate that Eclipse is still the preferred development environment for almost half of Java developers. Eclipse is the Swiss army knife of IDEs, featuring a heavily customizable interface and countless plugins. It’s particularly popular among enterprise developers due to it’s large developer community and extensibility. And in fact, it’s so ubiquitous that all of the other tools in this post provide Eclipse plugins.
Eclipse separates its workflow into three areas: the Workbench, the workspace, and the perspective. The Workbench acts as the starting point to the IDE. Workspaces group projects, files, and configuration settings under a single directory. Perspectives define the tools, views, and settings available in the Workbench based on the context of the workspace. Although new developers might find it more difficult to use than Netbeans or IntelliJ IDEA, Eclipse’s flexibility makes it the preferred IDE for enterprise development.
Neon, the latest version of Eclipse, brings support for high DPI monitors on Windows and Linux, a new Smart Import wizard for generating an Eclipse project around existing source code, improved editing tools, improved JavaScript and JSON tools, and support for PHP 7.
Gradle
Gradle is a project automation tool that builds on the features of Apache Maven and Apache Ant. While Gradle isn’t the most popular build tool available (that honor goes to Maven, which 68% of Java developers prefer), it’s quickly gaining popularity. It also serves as the default build tool for Android.
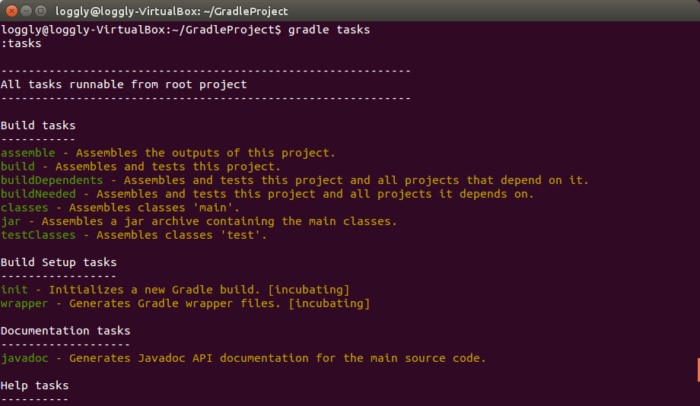
Gradle prides itself on simplicity. Gradle uses the Groovy programming language as opposed to the XML syntax used by Maven and Ant. A basic Gradle build file for a Java project consists of a single line:
apply plugin: 'java'.
The following command generates a Gradle build file, creates a directory tree for project files, and bundles a portable Gradle wrapper with the project:
$ gradle init --type java-library
Gradle includes additional plugins for adding new languages, generating project files for IDEs, building native binaries, checking for updated dependencies, and more.
For more information, see the Gradle Getting Started guide for Java/JVM.
Javadoc
Javadoc is a documentation generator provided by Oracle. It parses specially formatted comments into HTML documents. This screenshot shows the Java SE 8 API specification as generated by Javadoc:

Javadoc comments are formatted using an opening tag, a closing tag, and one or more descriptive tags. The opening tag is similar to the standard Java multi-line comment tag, except it uses two asterisks. Javadoc also parses plain HTML tags.
Javadoc automatically formats tags and keywords unless otherwise specified. Javadoc also makes extensive use of hyperlinks, allowing you to reference and link to separate areas of code. Many IDEs—including Eclipse—can automatically add Javadoc comment blocks to variables, classes, and methods. Plugins for Maven, Gradle, and Ant build Javadoc HTML along with your compiled code. For more information, see the Oracle article How to Write Doc Comments for the Javadoc Tool.
JUnit
JUnit is an open source framework for writing and running unit tests. A basic JUnit test consists of a testing class, a testing method, and the functionality to be tested. JUnit uses annotations to determine how tests are structured and ran. For example, if your program has a class called MathClass with methods for multiplication and division, you can create JUnit tests to check for unexpected values. When passing the numbers 2 and 5 to the multiplication method, you’d expect to receive 10 as a result. When passing 0 as the second parameter to the division method, you’d expect an ArithmeticException to be thrown due to a division by zero:
The @Test annotation specifies that the method is a test case. JUnit provides additional annotations such as @Before, which you can use to set up the environment before the test runs. JUnit also lets you set Rules that define how the test methods behave. For example, the TemporaryFolder rule causes files or folders created by a test to be deleted once the test finishes.
For more information, see Getting Started with JUnit. Vogella also provides a tutorial on unit testing with JUnit.
Cobertura
Cobertura, a jcoverage fork, analyzes Java code for test coverage. Cobertura generates HTML-based reports based on the amount of code that isn’t covered by testing.
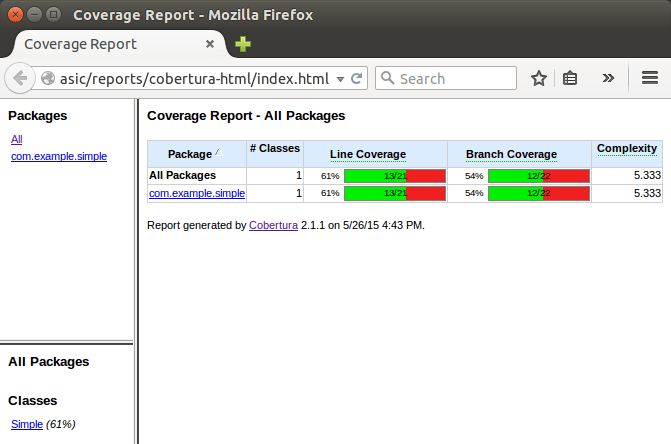
Cobertura provides tools for instrumenting, checking, and testing code. By monitoring instrumented code, Cobertura allows you to use your testing framework of choice or even run the program without a testing framework.
Cobertura reports code coverage in terms of lines, branches, and packages. Each category has a customizable threshold that triggers a warning if coverage falls below the threshold. Cobertura also integrates with Maven and Gradle for automatic detection.
Mkyong.com provides an example for integrating Cobertura with Maven.
FindBugs
FindBugs is a tool that pattern-matches compiled code against a database of bugs. When provided with the source code, FindBugs also highlights the lines of code affected by detected bugs.

As of version 3.0.1, FindBugs maintains hundreds of bug descriptions. Bugs are categorized into four levels based on their severity: of concern, troubling, scary, and scariest. In addition to a GUI, FindBugs provides a command-line interface, an Ant task, and an Eclipse plugin.
VisualVM
Included in the JDK, VisualVM is a utility for monitoring and reviewing the performance of Java applications. VisualVM detects and attaches to active JVM instances to retrieve diagnostic information about a process.

VisualVM makes it easy to diagnose performance issues in real time. It provides a full suite of profiling tools including JConsole, jstack, jmap, jinfo, and jstat. Additionally, you can take a snapshot of a JVM at any time for later review.
Groovy
Groovy is a programming language that both simplifies and extends Java by adding new keywords, auto-importing commonly used classes, and optionally typed variable declarations.
One of Groovy’s core strengths is its scripting capabilities. Classes can be compiled as Java bytecode or executed dynamically using the Groovy Shell. Groovy’s Java base makes it more accessible to Java developers when compared with Jython or JRuby.
For more information, see the Groovy Getting Started guide.
Honorable Mention: IntelliJ IDEA
Like Eclipse, IntelliJ IDEA is a multi-purpose IDE with a focus on Java development. IntelliJ IDEA has seen a huge boost in popularity among Java developers, and in 2016 actually overtook Eclipse as the most popular Java IDE due in part to its accessible interface and code analysis features. IntelliJ IDEA also forms the base of Android Studio – Google’s Android IDE – after replacing the Android Development Tools plugin for Eclipse.
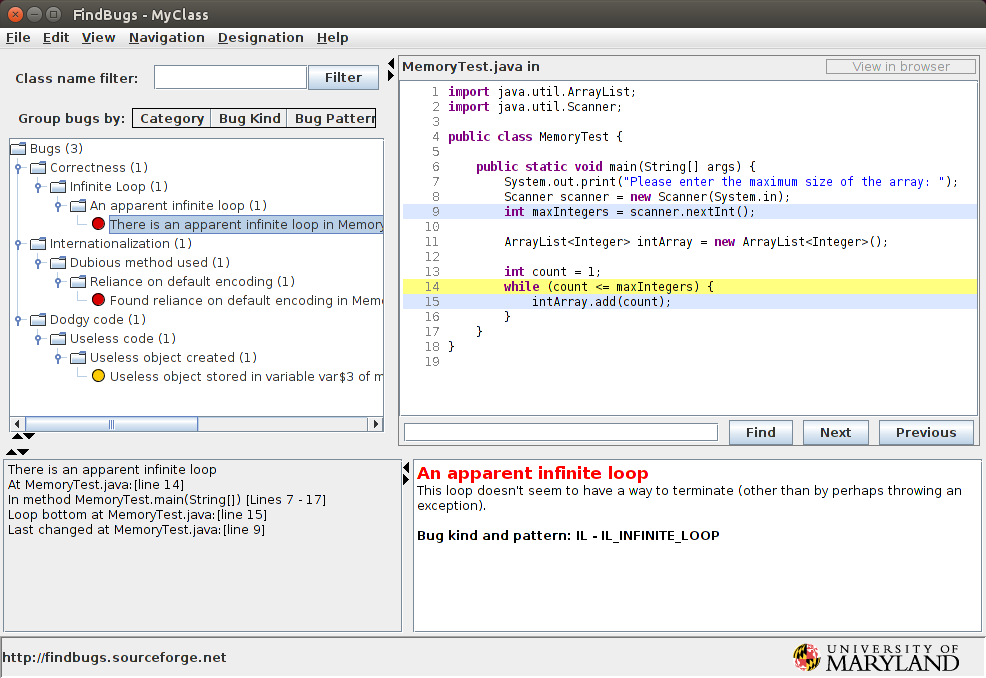
IntelliJ IDEA comes in two editions: the free Community Edition, and the subscription-based Ultimate Edition. The Community Edition supports Java and several Java-based languages, Android development, support for popular build tools such as Maven and Gradle, and built-in version control tools. The Ultimate Edition adds greater support for web development, database tools, and frameworks such as Spring and GWT.
Other Options
The Java landscape is constantly changing with new tools, utilities, and libraries. If your preferred tool didn’t make the list, feel free to share it in the comments below.
Additional Reading
Andre Newman is a software developer and writer on all things tech. With over eight years of experience in Java, .NET, C++, and Python, Andre has developed software for remote systems management, online retail, and real-time web applications. He currently manages 8-bit Buddhism, a blog that blends technology with spirituality.
The Loggly and SolarWinds trademarks, service marks, and logos are the exclusive property of SolarWinds Worldwide, LLC or its affiliates. All other trademarks are the property of their respective owners.
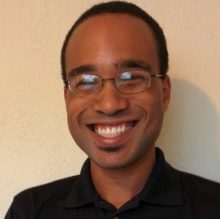
Andre Newman